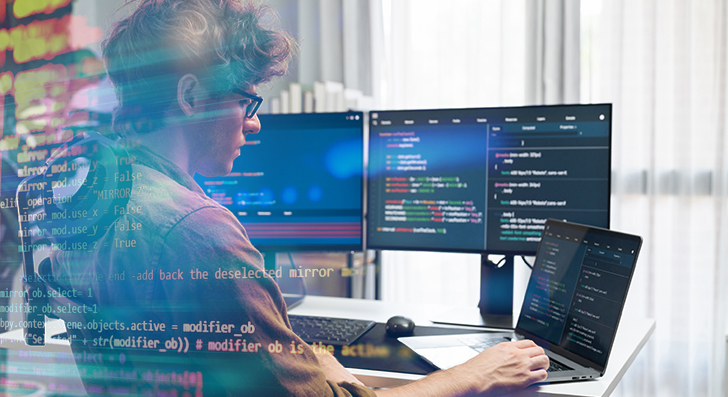
Scalability implies your software can cope with progress—much more buyers, additional info, and even more visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and worry later on. Here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability just isn't some thing you bolt on afterwards—it should be section of the approach from the beginning. Lots of programs fail every time they expand speedy since the first design and style can’t tackle the extra load. For a developer, you have to think early about how your procedure will behave under pressure.
Start out by designing your architecture to get flexible. Stay away from monolithic codebases where by anything is tightly connected. As an alternative, use modular structure or microservices. These patterns split your application into lesser, independent elements. Each individual module or provider can scale By itself with out impacting The full procedure.
Also, consider your database from working day a person. Will it need to manage one million users or perhaps 100? Select the suitable style—relational or NoSQL—according to how your info will increase. Strategy for sharding, indexing, and backups early, Even though you don’t need to have them still.
A further important stage is in order to avoid hardcoding assumptions. Don’t publish code that only will work underneath existing problems. Contemplate what would transpire In the event your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use layout styles that support scaling, like message queues or party-pushed units. These assistance your application cope with additional requests devoid of getting overloaded.
When you build with scalability in your mind, you are not just planning for success—you're reducing upcoming problems. A very well-prepared process is simpler to maintain, adapt, and grow. It’s better to arrange early than to rebuild later on.
Use the correct Database
Deciding on the right databases can be a crucial part of setting up scalable apps. Not all databases are developed exactly the same, and utilizing the Erroneous one can sluggish you down or even induce failures as your app grows.
Begin by being familiar with your knowledge. Is it really structured, like rows in the table? If yes, a relational databases like PostgreSQL or MySQL is an effective fit. These are definitely sturdy with relationships, transactions, and regularity. They also assist scaling methods like examine replicas, indexing, and partitioning to manage much more website traffic and info.
In the event your info is a lot more versatile—like person activity logs, product or service catalogs, or documents—consider a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with substantial volumes of unstructured or semi-structured information and might scale horizontally more simply.
Also, take into consideration your go through and produce patterns. Are you presently carrying out numerous reads with much less writes? Use caching and read replicas. Do you think you're managing a hefty publish load? Take a look at databases that may take care of higher publish throughput, or simply event-primarily based knowledge storage devices like Apache Kafka (for temporary information streams).
It’s also sensible to Assume in advance. You might not need to have State-of-the-art scaling features now, but choosing a database that supports them implies you gained’t need to have to change afterwards.
Use indexing to hurry up queries. Prevent needless joins. Normalize or denormalize your info determined by your entry styles. And generally observe databases general performance when you mature.
In short, the right database is determined by your app’s construction, speed needs, and how you expect it to grow. Take time to select sensibly—it’ll help you save a lot of trouble afterwards.
Improve Code and Queries
Rapidly code is vital to scalability. As your app grows, each small hold off provides up. Badly created code or unoptimized queries can slow down overall performance and overload your method. That’s why it’s important to build economical logic from the beginning.
Commence by writing clean up, uncomplicated code. Keep away from repeating logic and remove anything pointless. Don’t pick the most intricate Remedy if a simple just one performs. Keep your capabilities quick, focused, and simple to test. Use profiling applications to uncover bottlenecks—spots exactly where your code usually takes way too lengthy to operate or makes use of too much memory.
Upcoming, examine your databases queries. These usually gradual points down over the code alone. Ensure each query only asks for the info you really have to have. Stay away from Find *, which more info fetches every little thing, and instead decide on certain fields. Use indexes to hurry up lookups. And steer clear of executing a lot of joins, Specifically throughout large tables.
In case you notice the identical facts becoming requested time and again, use caching. Keep the effects temporarily making use of instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your database operations once you can. In place of updating a row one after the other, update them in groups. This cuts down on overhead and can make your application more effective.
Remember to examination with substantial datasets. Code and queries that do the job fine with 100 information may possibly crash if they have to take care of one million.
In brief, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when wanted. These techniques assistance your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to handle much more consumers and even more targeted traffic. If almost everything goes by way of one particular server, it can promptly turn into a bottleneck. That’s the place load balancing and caching are available in. These two resources support maintain your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As an alternative to one particular server carrying out each of the function, the load balancer routes users to different servers dependant on availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other folks. Equipment like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it could be reused rapidly. When buyers ask for the exact same details again—like an item webpage or a profile—you don’t should fetch it from your databases whenever. You are able to provide it from your cache.
There's two widespread kinds of caching:
1. Server-aspect caching (like Redis or Memcached) stores facts in memory for quick obtain.
2. Customer-side caching (like browser caching or CDN caching) outlets static files near to the user.
Caching lowers databases load, enhances velocity, and would make your app far more efficient.
Use caching for things that don’t improve usually. And normally ensure your cache is current when information does adjust.
In short, load balancing and caching are basic but impressive resources. Jointly, they assist your application handle far more buyers, stay rapidly, and Get better from problems. If you intend to improve, you need the two.
Use Cloud and Container Instruments
To make scalable applications, you will need instruments that permit your app increase conveniently. That’s where cloud platforms and containers are available in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Providers (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to lease servers and expert services as you would like them. You don’t have to purchase components or guess long run potential. When targeted visitors improves, you can add more resources with just a few clicks or immediately utilizing auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and security tools. You'll be able to concentrate on developing your app in lieu of running infrastructure.
Containers are A different critical Device. A container packages your app and all the things it ought to operate—code, libraries, settings—into one device. This causes it to be straightforward to move your app concerning environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application makes use of multiple containers, applications like Kubernetes allow you to control them. Kubernetes handles deployment, scaling, and Restoration. If just one element of your application crashes, it restarts it immediately.
Containers also allow it to be straightforward to individual parts of your application into solutions. You could update or scale areas independently, that is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment means it is possible to scale quick, deploy quickly, and recover speedily when issues happen. If you need your application to expand without the need of limitations, start out utilizing these instruments early. They save time, minimize hazard, and assist you to keep centered on developing, not repairing.
Watch Every thing
In case you don’t observe your application, you gained’t know when things go Improper. Checking allows you see how your app is executing, place challenges early, and make much better choices as your application grows. It’s a critical Element of developing scalable techniques.
Start out by monitoring essential metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and companies are executing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you acquire and visualize this facts.
Don’t just observe your servers—observe your application too. Keep an eye on how long it will take for customers to load webpages, how often mistakes occur, and in which they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Setup alerts for essential issues. For instance, When your response time goes over a limit or a service goes down, you should get notified straight away. This allows you deal with difficulties rapidly, usually just before customers even notice.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it again ahead of it leads to real problems.
As your app grows, traffic and facts boost. With out checking, you’ll skip indications of difficulties till it’s much too late. But with the best resources set up, you remain on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about knowing your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even small apps have to have a powerful Basis. By designing meticulously, optimizing sensibly, and using the suitable tools, it is possible to build apps that improve smoothly without having breaking stressed. Start tiny, Imagine large, and Create intelligent.